Hey Coders, Today’s going to be fun tutorial. Because Today, I’m going to give you A full fledged Guide that How To Send An Email using Python scripts. All the code and modules are explained below that will help you to sending emails using python.
To send an Email in Python, First you need to import some classes to send an email using SMTP servers. Simple Mail Transfer Protocol(SMTP) is basically a communication protocol to transfer electronic mails.
Sending Emails Using Python (Tutorial):
Basically In Python Standard Library, We have a package called “email” that will be help you to send emails using Smtp server through you Python script.
First, We will import this following code in our python file:
from email.mime.multipart import MIMEMultipart
In this above code, We have imported a class called MIMEMultipart from sub-packages of “email” package. Here, Under Email package, we have mime package that stands for Multipurpose Internet Mail Extensions that used to give format to your Emails.
First, Let me show you my code and after then I’ll define each and every line briefly. By the end of this article, You will have full knowledge about sending emails using python.
Python Email Send.py
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
import smtplib
message = MIMEMultipart()
message["from"] = "Hulas Karn"
message["to"] = "[email protected]"
message["subject"] = "Enter Subject of your Email"
message.attach(MIMEText("Type the message that you want to send to user"))
with smtplib.SMTP(host="smtp.gmail.com", port=587) as smtp:
smtp.ehlo()
smtp.starttls()
smtp.login("[email protected]", "password")
smtp.send_message(message)
print("You Message Sent")
Now, I’m going to explain each line of code separately.
Line_1: First, We have imported our MIMEMultipart class from email package.
Line_2: Then we have MIMEText class, that will help to attach body of our email.
Line_3: Imported smtplib module, that will help to establish connection with smtp server.
Line_5: Here, we have created an object called message of MIMEMultipart(). In this class, we have some defined headers e.g from, to, subject.
Line_7: Here, we declared a header of message object, where you need to enter Sender Name. To declare this header, you need to define it as message[“from”] = “Your Name Or Sender Name”.
Line_8: After then we need to define message[“to”] = “receiver email address”. In this header, you need to enter receiver’s email address.
Line_9: This header will define subject part of your Email. You need define it as message[“subject”] = “subject of your body”.
Line_10: Here, We don’t have any header to define body of our mail. Therefor, we have a to use attach() function. Under this function, we will use MIMEText() class to define our body of mail. This MIMEText() comes from Line 2, where we have defined sub-package from mime module.
To define body of you email, you need to use this code:
message.attach(MIMEText(“Your Message”))
Line_12: Here, you need to create an object from smtplib class that we had imported at line 3. Because this will help us to establish connection with smtp mail server.
But here, we will be use “with” statement because Once the message would be sent then we need to close our connection. Therefore, instead of creating object separately and closing it, we will use “with” statement.
If you don’t familiar with “with” statement, then you could also create an object of smtp e.g:
SMTP object Without “With” Statement:
smtp = smtplib.SMTP(host="smtp.gmail.com", port=587)
----
----
smtp.close()
But In Line 12, I’ve used “with” statement to create object of smtplib class. In this statement you have to enter two fields – host and port.
Host and port no. will be depend upon the server that you are using. Because, we’re using gmail service therefore host=”smtp.gmail.com” and port=587.
Line_13: Here, We’ve called ehlo() function of smtplib class e.g smtp.ehlo(). It tells the server that we’re client and we want to send an email.
Line_14: smtp.starttls() ensure TLS security for mails, which means Transport Layer Security.
Line_15: smtp.login(“sender_email”,”sender_password”) – In this function, you need to enter two fields, first one Your or Senders email address and password of your email.
Line_16: smtp.send_message(message): In this function, You need to enter MIMEMultipart object that you declared at Line 5.
Line_17: Now, we simply print a message e.g print(“message sent”), which help us to determine that our message sent successfully.
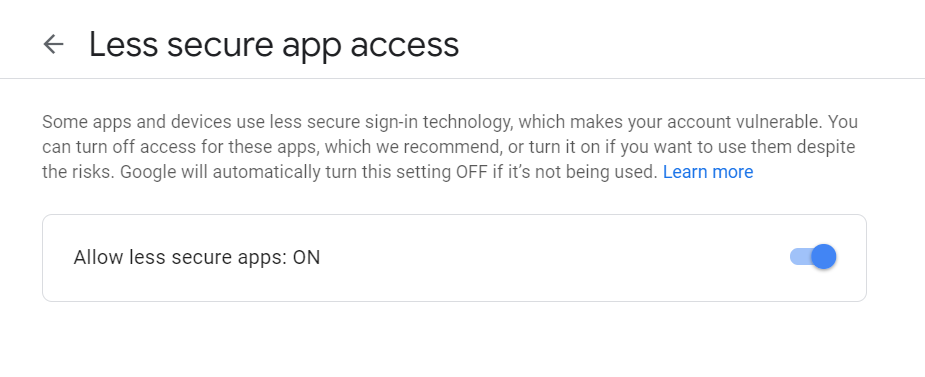
Or
If you have Two step verification then you need to create an app password.
Once you’ve done all above process then just run your program and you will be see “Your message sent” on terminal.
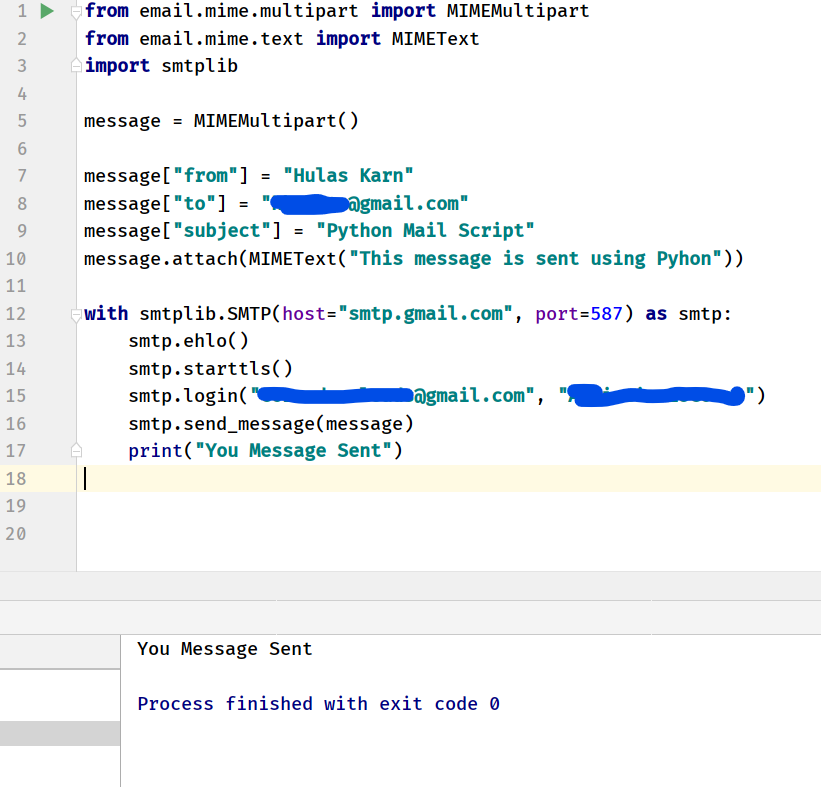
Now open receivers gmail account and check emails, where you will be see that message sent by Python script.
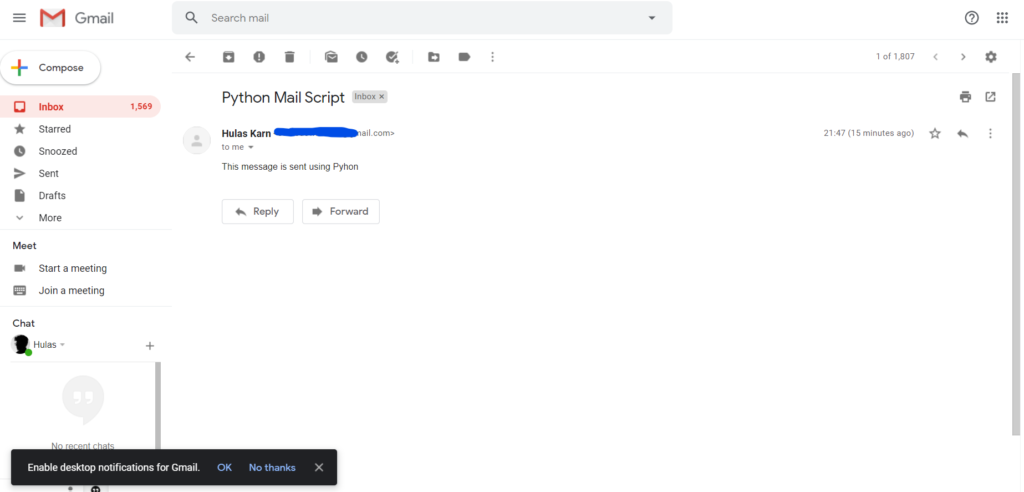
You May Also Like: FULL GUIDE TO BUILD AUTHENTICATION APP IN DJANGO
These were the basic structures to sending emails using Python Language. I hope you guys enjoyed this. Please don’t forget to share this article with your friends and Programmers.
Thank’s To Read…
1 thought on “Sending Emails Using Python Script(GUIDE)”