Hi everyone, I hope you are doing good. So, I know it’s been a long time. But now I am here with something really interesting. In today’s article, I am going to tell you how you can use OpenAI API, so that you can build your own ChatGPT with help of that.
But first let me list what we’re going to do:
- We will start with very basic explanation of APIs that we’re going to explore.
- And then will see how to use them.
For this article, we will use node.js environment. So without further ado lets start.
Why OpenAI API?
OpenAI has lot of useful ML models already trained on billion and trillions of parameters, and it gives you an oppurtunity to use those models through an API service that it provides. Although there are lot of useful apis available for different models. But we will mainly focus on text completion and chat completion APIs.
Pricing
But before that lets try to understand the the pricing model of these models that can be found in OpenAI Pricing page.
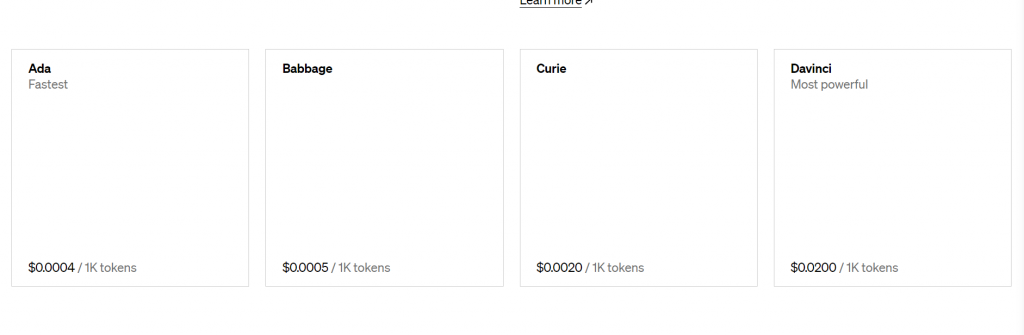
Although prameters of most of the models are same but there are some difference in the way we pass parameters between text and chat completion apis.
Let’s see the pricing of the models that we will use:
- 0.02$/1k tokens [text-davinci-003] (very efficient and accurate model just like GPT 3) [Part of Text Compeletion API]
- 0.002$/1k tokens [gpt-3.5-turbo] (for chat like interface and also cost effective) [Part of Chat Completion API]
How to use OpenAI API?
In order to use these APIs, you need to have an API key of OpenAI. And to get that key you need to have an account at OpenAI. So, just create one if you don’t have that, you can signup for openai account here: Signup for OpenAI
Once you signed up, after that you need to create an API key, you can do that by clicking on your profile icon and then click on View API keys option. Here click on create_secret_key and then enter any nickname for it. And after that it will generate an API key for you just copy it right now and store it somewhere. Because you will not be able to see that api key again… Only way to get an API again is by deleting and generating new one.
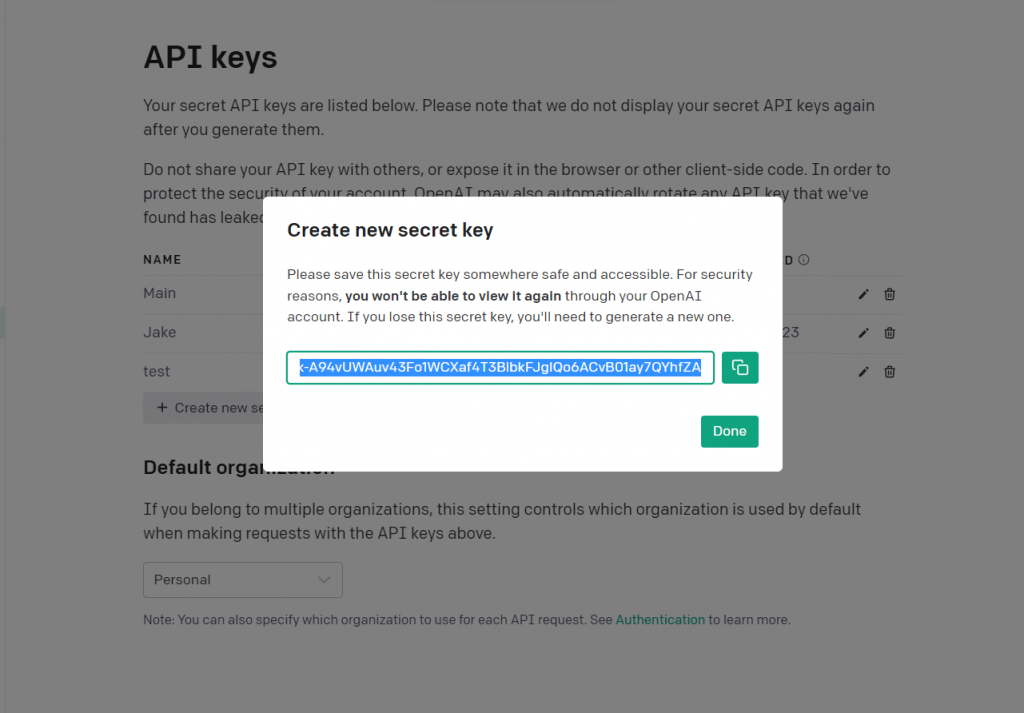
Setup Node.js Project:
Now setup your node.js project where we will test our api endpoints. To do so, you can use following commands one by one in your terminal:
npm init -y
and then
npm install openai
Give your project a name and its ready. Now create a new file called index.js this will be the place where we will write and test our api key. Open this project in any IDE you want and open index.js file.
Text Completion API:
We have 4 models in text-completion apis that are following:
- ada
- babbage
- curie
- davinci
Out of which, davinci is most powerful and these are basically very useful when you want do some instructional sort task but not only limited to that. We will see how to use text-davinci-003 model also called davinci in short. And rest of the models you can use in the same way… because they all follow the same format.
But before that, lets try to understand different parameters that we have:
- max_tokens: It’s a way to define how long your response can go upto.
- prompt: It’s an actual question or text that you will give to model(Kind of query) and on the basis of this it will return a response.
- model: It’s a place where you will define your model, model that you will use e.g. text-davinci-003, text-ada-002, etc.
- temperature: you have an option to set this from 0 to 10, basically it tells how creative it can be when generating a response, if it sets to 0 then response will be almost same every single time.
Now add the following code in your index.js file:
const { Configuration, OpenAIApi } = require("openai");
const configuration = new Configuration({
apiKey: 'xxx_api_key',
});
const openai = new OpenAIApi(configuration);
openai.createCompletion({
model: "text-davinci-003",
prompt: "Give me 15 different things related to blockchain in array like format:",
max_tokens: 50,
temperature: 0,
}).then(res => {
console.log(res.data)
})
Here is one thing to make sure that combined length of prompt and max_tokens should not be greater than in case of text-davinci-003 model. Because thats the limit you can’t go more than that in case of this model. And also change xxx_api_key with your own api_key .Here above you can see that I entered Give me 15 different things related to blockchain in array like format and then it gave this in return
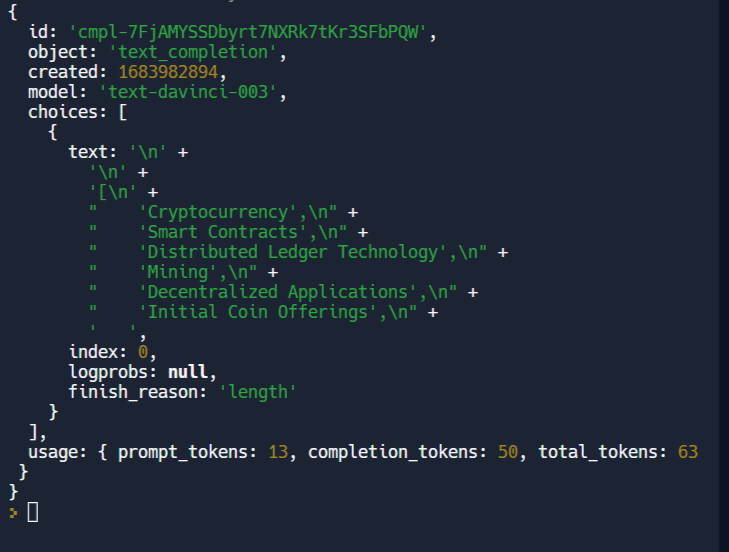
Where you can clearly see our response is avaiable at res.data.choices[0].text. And also returned an usage that show amount of tokens that we have used. So, thats how you can use text completion api of an Open AI.
Chat Completion API:
Although its also similar like text completion api but slightly different in terms of parameters, let’s see how.
In chat completion api, instead of prompt that we were directly passing as prompt parameter, we have a new parameter called messages
.
- messages: messages is consists of an objects, where each object has at least two properties: role and content.
- role: We have 3 types of role:
- system: where you will specifiy how the model should act e.g you are an intelligent doctor or you are a technical architect etc. But it’s completely optional.
- user: this can be considered as a prompt, this is the question that user will ask.
- assistant: Generated responses are given assistant role.
- content: It’s basically the value field of the that object, where you will define prompt value, system instruction or you will have your response as per the role defined.
- role: We have 3 types of role:
Okay so, now lets see how we have coded this.
const { Configuration, OpenAIApi } = require("openai");
const configuration = new Configuration({
apiKey: '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',
});
const openai = new OpenAIApi(configuration);
openai.createChatCompletion({
model: "gpt-3.5-turbo",
messages: [{role: "user", content: "Who are you"}],
}).then((res) => {
console.log(res.data.choices)
})
Okay so in above code, we’re using gpt-3.5-turbo model. and we have defined messages, where we have one object consists of role as an “user” and content is “who are you”. And after running this we got this in return.
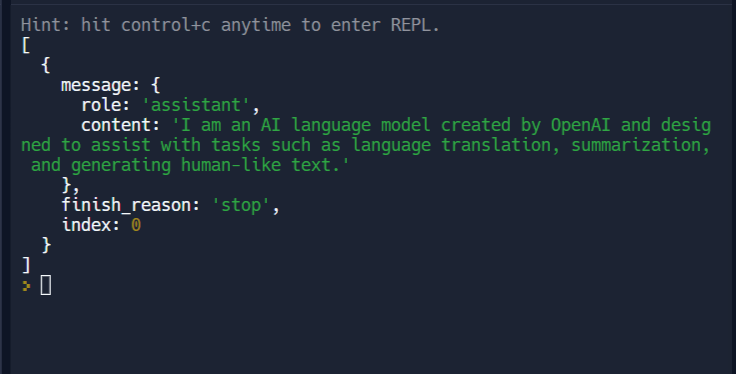
Here, we have printed res.data.choices and inside that we have one message section, where our response resides. this response has role as an “assistant” and then result inisde content section.
Here one this to note, to improve this code, you can store value of messages parameter somewhere upper, and at each response you can push this new message inside that messages section. In this way, you will proper context and sequence of chat that is going on, something like this:
const { Configuration, OpenAIApi } = require("openai");
const configuration = new Configuration({
apiKey: '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',
});
const openai = new OpenAIApi(configuration);
const messages = [{role: "user", content: "Who are you"}]
openai.createChatCompletion({
model: "gpt-3.5-turbo",
messages,
}).then((res) => {
messages.push(res.data.choices[0].message)
console.log(messages)
})
And then our response will be in proper chat like format:
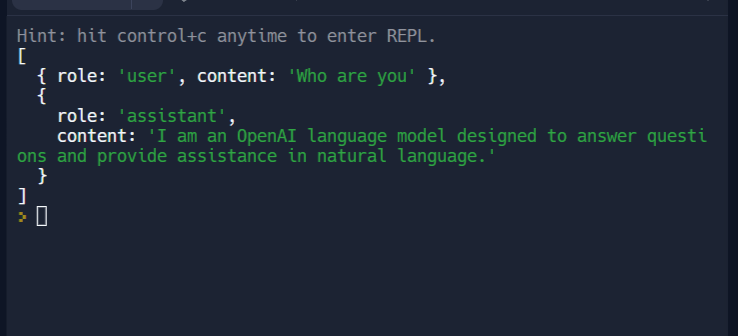
You may also like: Learn to use one of largest publicly available database called WikiData
So ya, that’s how you can use Open AI APIs, it was really easy right, isn’t it. So, if you liked this article then don’t forget to share this your friends.
Thanks to read