Hello coders, In this article, I’m going to show you that how you can create components in VueJS 3. But before that, let’s talk a little bit about component in VueJS 3.
What is a component?
Component is kind reusable code and that you can use throughout you Vue Application. And each component contains Its own data, methods, etc.
There are two way of registering component in Vue.
- Local Registration: In this, you can import component inside particular component. And then that component will be only accessible to this particular component.
- Global Registration: Here, you have to define your component globally inside the main.js file. So, that all component could access that particular component without importing it.
Now let’s start our guide about How to create component in Vue JS 3.
Creating and Registering Component Locally:
First of all, create a boilerplate of Vue app by following command.
vue create app_name
And then select Vue 3 to procced.
Once your project is created then simply run it. By default you get HelloWorld component. So, just delete it and also remove its import from App.vue file.
<template>
<div>
<!-- -->
</div>
</template>
<script>
export default {
name: 'App',
components: {
//component
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
Now we will create our component from scratch.
So, just create new HomePage.vue inside your src/component.
<template>
<div>
<h1>{{ name }}</h1>
</div>
</template>
<script>
export default {
data() {
return {
name: "CodeChit"
}
}
}
</script>
Now you can import this HomePage component inside other component. But first you have to import it. Here, we will import it locally.
Inside you App.vue, import HomPage.vue inside script tag. and register that imported component in components object.
<template>
<div>
<HomePage />
</div>
</template>
<script>
import HomePage from './components/HomePage';
export default {
name: 'App',
components: {
HomePage
}
}
</script>
Now you can render your <HomePage/> component in you App.vue file. Because we imported it here locally. You can also give name to your HomePage.
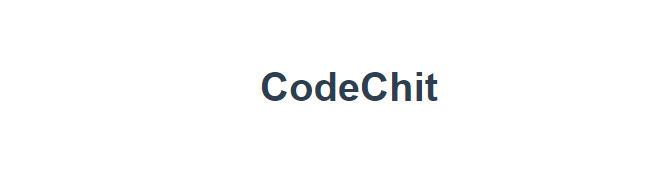
<template>
<div>
<home-page></home-page>
</div>
</template>
<script>
import HomePage from './components/HomePage';
export default {
name: 'App',
components: {
'home-page':HomePage
}
}
</script>
Registering Component Globally:
Now let’s create a component and register it globally. So, first of all, as usual, you have to create a Vue file. Let’s create this time Button component. and then we will register it globally. So that all other components could access it without importing it.
First create you Button Component:
<template>
<div>
<button>Click Here</button>
</div>
</template>
<style>
button {
padding: 10px 30px;
background-color: aliceblue;
outline: none;
border: 1px solid #333;
}
</style>
Then inside you main.js file first store your createApp function in a separate app variable then mount it. And after that import your Button component. and Then register your component using component method
app.component(‘tag’, component-name)
import { createApp } from 'vue'
import App from './App.vue'
import Button from './components/Button'
const app = createApp(App)
app.component('button-comp', Button)
app.mount('#app')
Now you can use this <button-comp> to render this component inside any component without importing it.
<template>
<div>
<h1>{{ name }}</h1>
<button-comp></button-comp>
<br>
</div>
</template>
<template>
<div>
<home-page></home-page>
<button-comp></button-comp>
</div>
</template>
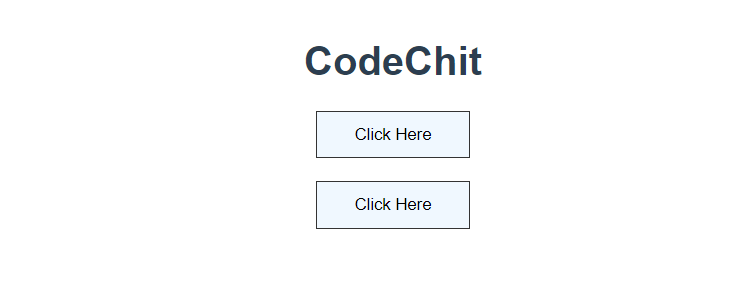
Props: Passing Data in component:
You can also pass any data to these components and then later you can receive these data into that component using props.
Lets pass msg data to <home-page> and then we will receive this msg variable inside HomePage component using props.
<template>
<div>
<home-page msg="It's a message"></home-page>
<button-comp></button-comp>
</div>
</template>
<template>
<div>
<h1>{{ name }}</h1>
<p>{{ msg }}</p>
<button-comp></button-comp>
<br>
</div>
</template>
<script>
export default {
props: ['msg'],
data() {
return {
name: "CodeChit"
}
}
}
</script>
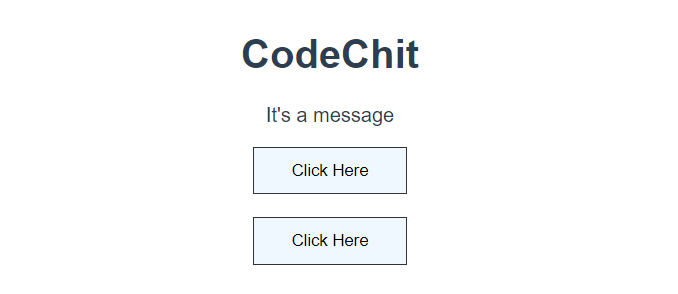
You May also like: VueJs 3 Starting Guide for Beginners 2021
So, I hope you all liked this article about component in VueJS 3. Please don’t forget to share this with your friends. You can also consider subscribing to our blog if you want to stay updated with such kind of articles.
Thanks to read…