Bubble Sort is one the most simplest sorting algorithm out there. And in this, we repeteadly go trough each item in the list and would compare adjacent items. If current item is bigger than next item then swap them[For ascending order]. Let me explain you this by diagram.
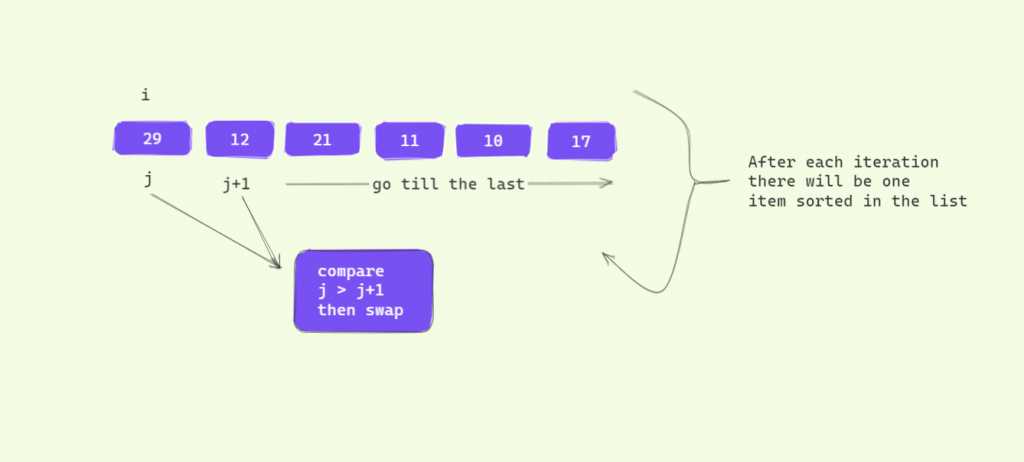
So, basically we loop through the the array and at each iteration we loop one more time(kind of nested loop). and nested loop goes upto the n-i-1
. where n is no of elements in the array, and i
is the iteration number of parent loop. Because at each iteration of parent loop there will be one item sorted in our list.
Inside nested loop, we compare current item arr[j]
with next item arr(j+1)
. And if current item(arr[j]) is greater than next item(arr[j+1]) then swap it. And you will have sorted list by end of parent loop.
Implementation In C++
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int N) {
int i, j;
for(int i = 0; i<N - 1; i++) {
for(int j = 0; j < N - i - 1; j++) {
if(arr[j] > arr[j+1]) {
swap(arr[j], arr[j+1]);
}
}
}
}
void printArray(int arr[], int N) {
for(int i=0; i<N; i++) {
cout<<arr[i] << endl;
}
}
int main() {
int arr[] {1,3,2,34,34,345345,332,2};
int N = sizeof(arr) / sizeof(arr[0]);
bubbleSort(arr, N);
printArray(arr, N);
}
Implementation in Javascript:
function swap(arr, i, j) {
let temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
function bubbleSort(arr) {
for(let i = 0; i<arr.length; i++) {
// start j at 0 and at each iteration 1 item from last will get sorted. So
// you can keep length in j after each iteration of i [i<n-i-1]
for(let j = 0; j < arr.length - i - 1; j++) {
if(arr[j] > arr[j+1]) {
swap(arr, j, j+1);
}
}
}
return arr;
}
console.log(bubbleSort([1,3,34,332,2]))
Although, its not that efficient if you would compare this with other algorithms out there. but still its good to know. I hope you liked this article if yes then please don’t forget to share this with your friends.
Thanks for reading 🙂