Map() function is one of the useful functions in Python. In this article, we’re going to explore what is Map function and when you can use this function.
Python map() function:
A map function is also an iterable object. Basically, the Map function takes two parameters: one is a function and then the second is an iterable_object. This is the syntax structure:
Map(func, iterable_object)
How does the Map() function work?
Basically, the Map function takes items from iterable_object and then passes them through the given function on each iteration, And then stores the result from each iteration inside the Map object. Now let’s try to understand it with an example.
for instance, let’s perform map function on list.
numbers = [1,2,3,4,5,6,6,7]
So, Now I want to multiply each items of numbers list from 3. One way to do this by using for loop, but that could be length process. But here we can use map() function to achieve the same result.
All we need a function, which should take an integer and then return multiplication of 3. and then the iterable object(which is numbers here).
numbers = [1,2,3,4,5,6,6,7]
def three_mutiply(num):
return num * 3
new_numbers = map(three_mutiply, numbers)
print(list(new_numbers))
[3, 6, 9, 12, 15, 18, 18, 21]
In the above, we defined the three_multiply function which takes one argument and then multiplies it by 3. Now you can pass it inside the map function and then your list(numbers). Now what it does, It takes one item(1) from the numbers list and then passes it through the three_multiply(1) function, and then the result will be stored inside the map object. It will perform the same task on every item from the numbers list.
You can store this map object inside separate variable. And now because map is an iterable object, so you can create list by using list() function
Using Lambda function inside Map function:
In the above code, we can make our code much simpler using Lambda Function. So, we all know that Map function takes one function as an argument. and basically that function takes one argument and return expression.
So, instead of defining def three_multiply(), we can actually use lambda function here to make it simple. e.g:
def three_mutiply(num):
return num * 3
Or
lambda num: num * 3
So, because we need this function only one time, that’s why using lambda expression would better and simpler.
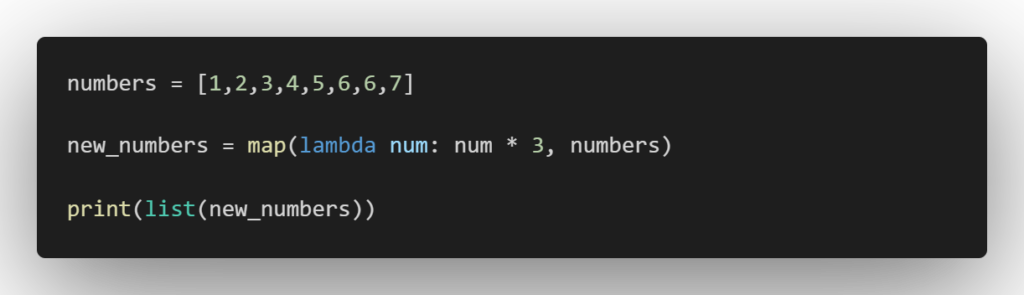
So, In simple words,
Map(func, iter) is an iterable object which takes one item from given iterable object and then passes it through given function. And then stores the result inside Map Object.
MAP Function
Read also: Python Basic: Lists and methods
I hope you all liked this article. So, please don’t forget to share this article with your friends and other geeks. You can also subscribe to our blog via email to get future notifications from this blog.
Thanks to read…
1 thought on “Map() function in Python: Python Basics”